I know this forum favors open source applications but since you are on windows I think a scheduled task running powershell might suit your needs with minimal installs/modifications. The task scheduler portion is the easy part so lets determine if powershell can do it before we set anything up.
Disclaimer: I’m only OK at Powershell - I won’t get defensive if anyone chimes in with a better way to do this because I’m sure there are many.
The website you listed prompts a download button.
Taking a peek via chrome devtools shows a button class.
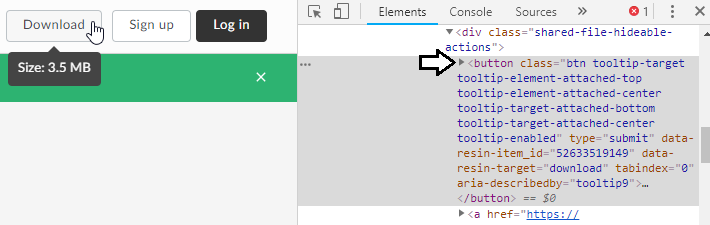
In .net there is are command to open an an IE window and work with it through powershell and other .net applications.
#Create an IE Object
$ie = New-Object -com InternetExplorer.Application
#Allow it to open an IE Window.
$ie.visible = $true
#Open the website in IE
$ie.navigate("https://cbaa.app.box.com/s/yemq5chyijnstu2foyzv2xucist56riw")
These commands should open an IE window.
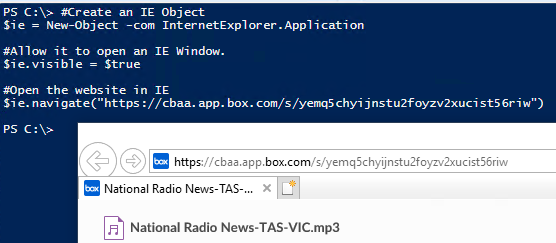
The variable $ie now contains the live Internet Exporer Window an object.
Further interactions can be performed with the variable in this powershell session.
You can output the object directly to see what it contains.
$IE
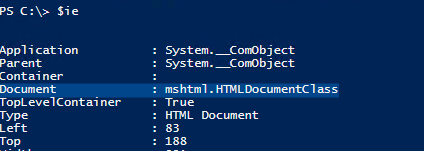
I wasn’t sure what look for so I expanded the properties until I found the page info in document.
If we try a standard select command then it just displays the type data.
$ie | select document
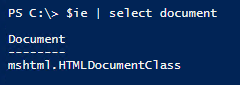
We want to expand this and treat it as its own object.
The easiest way I know to do this is to use a parenthesis.
($ie).document
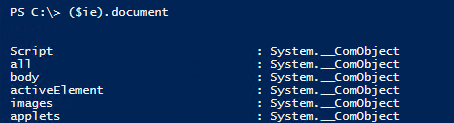
This gives us a ton of properties but did not allow me to filter by the"button class we found earlier in chrome. I googled a bit and narrowed what we want down to the .getElementsByTagName() function
$ie.Document.getElementsByTagName("Button")
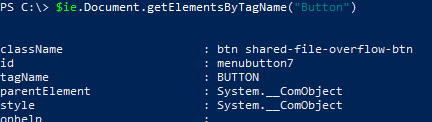
This command dumped less data out but button tag properties are still way to much to sort through. We need a way to narrow this down so we can find the right button.
Looking back at the Chrome Devtools we can see a data-resin-target property that contained “Download”.
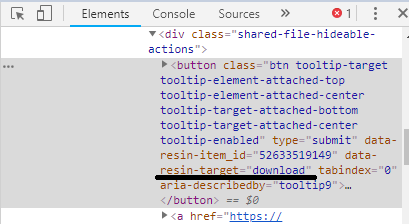
I tweaked with selecting properties until I found a way to output all the downloads.
Poking through the properties I determined that the Outerhtml property seems to contain a dump of related html. We should be able to output that by piping to select-object.
$ie.Document.getElementsByTagName("Button") | select outerhtml
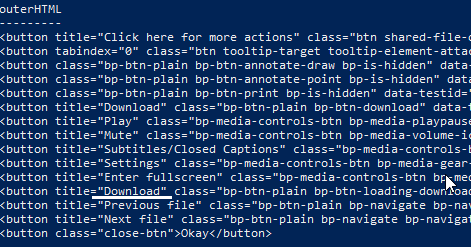
The underlined Download portion looks to be what we want so lets drop a where-object statement in the pipeline to filter this madness down a bit.
$ie.Document.getElementsByTagName("Button") | where{$_.Outerhtml -like "*Download*"} | select outerhtml

We are down 3 Responses now!
Download File looks like the best choice so lets adjust our where wildcard filter to that.
$ie.Document.getElementsByTagName("Button") | where { $_.outerhtml -like "*Download File*" } | select outerhtml
Bingo.
We are down to 1 result.
Powershell doesn’t care about what is in the object and will need to entire object to download the file so lets take out the select filter. With that removed we can the object pipe that into a .click() command that I found during my googling time. Some posts put the whole element grab into a variable but I’m just going to toss our filter into a parenthesis and put the .click() after it.
($ie.Document.getElementsByTagName("Button") | where { $_.outerhtml -like "*Download File*" }).click()
This command will execute on the IE window.
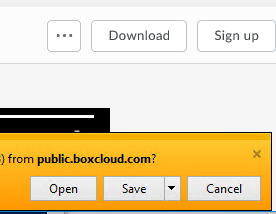
Partial Success! We now have a download prompt but it requires a manual click.
I need to walk away for a bit, but this may be enough to start tinkering on your own. I should have more time to look a this tonight.
The remaining tasks left are:
- To detemine a method of clicking save
- Tweak it so that it works without opening a window
- Setting up a scheduled task to run it.
Powershell Script so far:
#https://forum.level1techs.com/t/scripting-download-of-file-from-box-com-link/
#Tested on a machine using Windows 10 and powershell 5.1
#Powershell 5.1 download available here if needed.
#https://www.microsoft.com/en-us/download/details.aspx?id=54616
#Create an IE Object
$ie = New-Object -com InternetExplorer.Application
#Allow it to open an IE Window.
$ie.visible = $true
#Open the website in IE
$ie.navigate("https://cbaa.app.box.com/s/yemq5chyijnstu2foyzv2xucist56riw")
#Wait for the page to load.
#I added this later due a dely my machine had openingIE.
while($ie.ReadyState -ne 4) { start-sleep -s 10 }
#Grab the proper download button and click it.
#Note: Sometimes this doesn't work correctly, even with the 10 second wait when a window opens up, may have to look for an alternative.
$URL = ($ie.Document.getElementsByTagName("Button") | where { $_.outerhtml -like "*Download File*" }).click()